Increase your development productivity by taking advantage of our Robot API feature.
Starting with UiPath 8 you can control the execution of processes from your .NET application by integrating it with our WCF service.
Prerequisites: UiPathStudio.msi installed
Let's start by creating a new project in Visual Studio. The sample code presented in this article is developed using Visual Studio 2015, but any other version can be used.
After creating the project, just go in Solution Explorer, right click on your project's name and select Add service reference. A service reference enables a project to access a Windows Communication Foundation service.
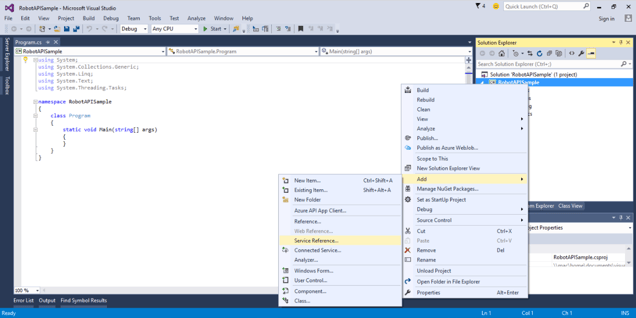
In the Address box, enter the address of UiPath Robot service, net.pipe://localhost/UiPath/service/MEX, and then click Go to search for it.
In the Namespace box, enter the namespace that you want to use for the reference.
Click Ok to add the reference to your project.
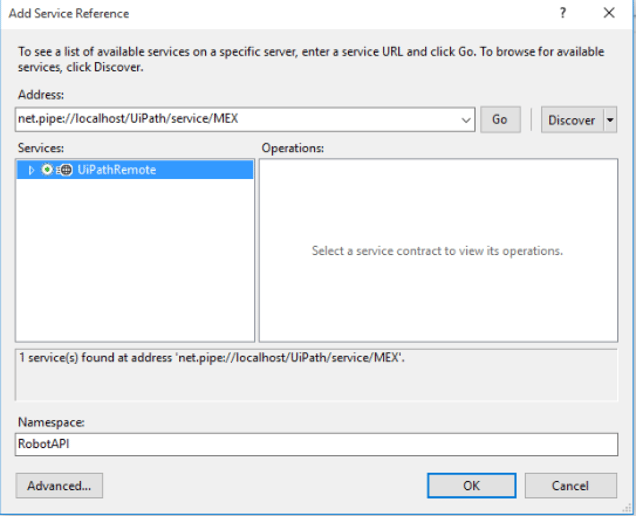
A service client (proxy) is generated, and metadata describing the service is added to the app.config file automatically.
All you need to do next is to create a class that handles your duplex communication and you're done. Below you can find a code sample.
[CallbackBehavior(ConcurrencyMode = ConcurrencyMode.Multiple, UseSynchronizationContext = false)] public class RobotClient : IUiPathRemoteDuplexContractCallback { private IUiPathRemoteDuplexContract Channel = null; private DuplexChannelFactory<IUiPathRemoteDuplexContract> DuplexChannelFactory = null; public RobotClient() { DuplexChannelFactory = new DuplexChannelFactory<IUiPathRemoteDuplexContract>(new InstanceContext(this), "DefaultDuplexEndpoint"); DuplexChannelFactory.Credentials.Windows.AllowedImpersonationLevel = System.Security.Principal.TokenImpersonationLevel.Impersonation; Channel = DuplexChannelFactory.CreateChannel(); } #region Service methods public Guid StartJob(string serializedJob) { return Guid.Parse(Channel.StartJob(SerializeStringToStream(serializedJob))); } #endregion #region Duplex callbacks public void OnJobCompleted(string invokeCompletedInfo) { var settings = new JsonSerializerSettings { TypeNameHandling = TypeNameHandling.None }; var completedResult = JsonConvert.DeserializeObject<InvokeCompletedArgs>(invokeCompletedInfo, settings); if(completedResult.State == System.Activities.ActivityInstanceState.Faulted) { Console.WriteLine(completedResult.Error.Message); } else if(completedResult.State == System.Activities.ActivityInstanceState.Canceled) { Console.WriteLine("Process cancelled"); } else { Console.WriteLine("Completed without errors"); } } #endregion Duplex callback }}
Main function
class Program { static void Main(string[] args) { var client = new RobotClient(); var job = @"{'WorkflowFile': 'C:\\Workflows\\RobotAPI\\Main.xaml', 'InputArguments': {'Message': 'Message from RobotAPISample'}, 'User': '<username>', 'Type': 0}"; Console.WriteLine(client.StartJob(job)); Console.ReadLine(); } }
The complete sample can be dowloaded from here.
Given that the Robot API is no longer available, could you update or post a new article on how to Start a Job with the newer Orchestrator API ?
ReplyDeleteThanks for sharing information...nice blog
ReplyDeleterpa uipath training in hyderabad
I really enjoyed your blog Thanks for sharing and it was very usefully to me
ReplyDeleteRPA UiPath Online Training
RPA UiPath Training in Hyderabad
RPA UiPath Training in Ameerpet
This information is really awesome thanks for sharing most valuable information.
ReplyDeleteUipath Training Hyderabad
Online Uipath Training
Uipath Course Online
Uipath Training in Hyderabad
Uipath Online Course
RPA Uipath Training
Uipath Training in Hyderabad
RPA Online Training
Uipath Training
Uipath Online Training
Uipath Course
Uipath Training
Uipath Training in india
I really enjoyed your blog Thanks for sharing and it was very usefully to me
ReplyDeleteVisit us: Java Online Training Hyderabad
Visit us: Core Java Online Course
Visit us: java course
Thanks for sharing such a nice info.I hope you will share more information like this. please keep on sharing!
ReplyDeleteVisit us: dot net training
Visit us: Dot Net Online Training Hyderabad
Visit us: .net online training india