Note: The Robot API is no longer supported. We recommend using the Orchestrator API.
There are four ways how you can run a process in UiPath Studio 8
- From UiPath Studio
- From UiPath Robot Agent
- From command line
- Through a REST command
This article shows how to run UiPath 8 processes from external applications and remote systems. For UiPath Studio 7.5 guidelines, check this article.
UiPath 8 exposes a REST service endpoint which you can use to start a process. A simple API allows you to leverage UiPath execution power into your own application or trigger it remotely.
Want to start a process from your .NET application?
POST a command using a WebClient object or any REST third party library.
POST a command using a WebClient object or any REST third party library.
Want to integrate your Java application with UiPath?
Use any implementation of JAX-WS to consume our REST service.
Use any implementation of JAX-WS to consume our REST service.
Want to trigger a process from your MAC?
Use cURL to easily start the process.
Use cURL to easily start the process.
Configure UiPath Robot service
- Install UiPath Studio msi
- Go to Local Services and stop UiPath Robot service
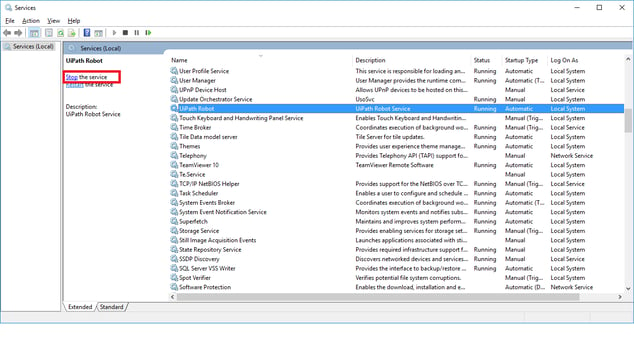
- Edit UiRobot.exe.config under UiPath installation folder and uncomment the REST endpoint
<!-- Enable this for UiPath Platform Preview support--> <!--<endpoint name="RestEndpoint" address="http://127.0.0.1:8080/UiPath/service/agent" binding="webHttpBinding" bindingConfiguration="WebHttpBinding_IRemoteContract" contract="UiPath.Models.IUiPathRemoteContract" behaviorConfiguration="RestEndpointBehaviour" /> <endpoint address="http://127.0.0.1:8080/UiPath/service/agent/mex" binding="mexHttpBinding" contract="IMetadataExchange" />-->
- Start UiPath Robot service
To easily test if the service is up and running just run Invoke-RestMethod command from PowerShell
Invoke-RestMethod http://127.0.0.1:8080/UiPath/service/agent/IsAlive -Method Get
By default, the service is hosted at http://127.0.0.1:8080 address. You can change this in UiRobot.exe.config file by changing the address of RestEndpoint.
UiPath Robot API
UiPath exposes a WCF service as a REST web service that you can consume from your application to start and manage processes. All methods have a JSON web message format.
All samples below can be executed in PowerShell 3.0 or higher.
Service Methods
bool IsAlive()
DescriptionGET method; Returns True if UiPath service is up and running.
RemarksUri template: service_address:port/UiPath/service/IsAlive
SampleInvoke-RestMethod service_address:port/UiPath/service/agent/IsAlive -Method Get
string StartJob(Stream processInfo) DescriptionPOST method; Starts the process defined by the JobModel passed. Returns a GUID that uniquely identifies the process started. RemarksProcessInfo parameter must be a JobModel object in JSON format Uri template: service_address:port/StartJob Sample$job ="{'WorkflowFile': 'C:\\Workflows\\Main.xaml', 'User': 'andra', 'Password': 'parola', 'Type': 0}"
$jobIdResponse = Invoke-RestMethod -Uri service_address:port/UiPath/service/agent/StartJob -Body $job -Method Post $jobId = $jobIdResponse.ChildNodes[0].InnerText
string QueryJob(string processId)DescriptionGET method; Retrieves execution information about the process identified by processId.
RemarksThe information represents a JobStatus object in JSON format.
Uri template: service_address:port/QueryJob/{processId}
SampleInvoke-RestMethod service_address:port/UiPath/service/agent/QueryJob/'{8be88bb4-3e51-40da-aca8-26d601be3c61}' -Method Get
void CancelJob(string processId)Description
GET method; Cancels the process identified by processId.
RemarksUri template: service_address:port/UiPath/service/CancelJob/{processId}
SampleInvoke-RestMethod service_address:port/UiPath/service/agent/CancelJob/'{8be88bb4-3e51-40da-aca8-26d601be3c61}' -Method Get
Service Models
JobModel
WorkflowFile | string | Represents the absolute path of the process; |
InputArguments | Dictionary<string, object> | A dictionary of input parameters keyed by argument name. |
User | string | Specifies the user account name that should execute the process. If this is not specified then CredentialTarget is mandatory |
Password | string | The Windows password of the user defined by User parameter. If this is not specified then CredentialTarget is mandatory |
CredentialTarget | string | The name of a Windows Credential that defines the user account that should execute the process. |
Type | int | Default 0. Available values {0,1}. If set to 0 then the process will start in an interactive Windows session of the user, otherwise it will start in session 0. |
SessionInfo | OpenSessionInfo | Defines if the Windows session should be monitored |
JobStatus
ExecutionStatus | string | Specifies the current status of the process. Available values {NotStarted, Processing, Finished, Error, Cancelled, Cancelling, JobMissing} |
ExecutionPoint | string | Represents the last faulted activity of the specified process. |
CompletedResult | string | The result of a finished process. It represents a InvokeCompletedArgs object in JSON format |
InvokeCompletedArgs
State | string | Describes the completed state of the process. More information about its values can be found here |
Error | Exception | Is set only when the process ends with error |
Output | Dictionary<string,object> | A dictionary of the Out and InOut values keyed by argument name that represent the outputs of the process |
Token | Guid | The id of the process |
WorkflowFile | string | Absolute path of the executed process |
Windows Communication Foundation Essentials(WCF)
Windows Communication Foundation (WCF) is a platform rich in features necessary for building distributed service-oriented applications. With WCF developers can create services that satisfy a vast number of scenarios for the intranet and Internet including classic client-server applications, services that match the simplicity of ASP.NET web services (ASMX), services that incorporate the latest web service standards (WS*), and services that support advanced security scenarios such as federated security. Designing and implementing services with WCF is not particularly difficult once you know the platform – therein lies the challenge. How can a developer get up to speed on the entire WCF platform quickly in order to be productive implementing WCF services? How can a developer new to WCF get up to speed on only those features they need to implement services that meet their application’s requirements? How can a developer be sure that they have not overlooked an important facet of WCF necessary to implementing their services in the best possible way?
Developers cannot be expected to have full command of the vastness of the WCF platform before designing and implementing services – but they can be expected to consider common scenarios and recommended settings for core features necessary to those scenarios. This whitepaper, broken into a series of topics, is intended to provide developers with concise guidance on WCF to reduce some of the noise created by the sheer number of features. Those new to WCF should read this whitepaper as a quick start guide to the features to at least consider during design and implementation of WCF services, and as specific scenarios are tackled. Those who already have experience with WCF can use this whitepaper as a checklist with recommendations to consider and things not to forget. Both audiences should see this as a way to make sure that sound choices are being made for contract design, hosting, configuration and the employment of extended WCF features.
Articles in this series
This section will serve as a summary of the steps you will take to design, implement, host and consume WCF services. The purpose is not to explain the details behind each design choice or feature – but to make you aware that these are things you should consider. Later sections of this whitepaper will provide recommendations to narrow the choices you face.
| |
This section summarizes the available project templates, when to use each; and discusses the limitations and benefits of the core WCF tools.
| |
This section will review core considerations for contract design including designing service contracts, choosing between complex type serialization formats, and when to use the XmlSerializer or message contracts.
| |
This section discusses how WCF contracts support backward compatibility, and explains a few versioning strategies that you might consider for your WCF applications.
|
Thanks a lot very much for the high quality and results-oriented help. I won’t think twice to endorse your blog post to anybody who wants and needs support about this area.
ReplyDeleteBest RPA Training in Bangalore
Nice and good article. It is very useful for me to learn and understand easily. Thanks for sharing your valuable information and time. Please keep updatingmulesoft online training
ReplyDeleteGreat Blog,
ReplyDeleteRPA Training in Hyderabad
RPA Training in Chennai
RPA Training in Bangalore
I really impressed in this article. very valid informative blogs and I learn easily understand.RPA Training Center in Chennai
ReplyDeleteNice work, your blog is concept oriented ,kindly share more blogs like this
ReplyDelete.NET Online Course Bangalore
the things you like about them and what you don't. Make a few notes on what you should need to emulate on your future site. Web design company Houston
ReplyDeleteUseful Information, your blog is sharing unique information....
ReplyDeleteThanks for sharing!!!
website designing Internships in hyderabad
best website designing companies in hyderabad
website designer in Hyderabad
web designing company in Hyderabad
Thanks for sharing wonderful information. Really Liked the content of this blog and the structure & design is also wonderful.
ReplyDeleteBlueprism online training
Nice Blog..... Keep Update.......
ReplyDeleteCustom apllication development in chennai
UI path development in chennai
rpa development in chennai
Robotic Process Automation in chennai
erp in chennai
Rpa Training in Chennai
ReplyDeleteRpa Course in Chennai
Blue prism training in Chennai
This article looks great thanks for the information.
ReplyDeleteAngular JS Training in Chennai | Certification | Online Training Course | Angular JS Training in Bangalore | Certification | Online Training Course | Angular JS Training in Hyderabad | Certification | Online Training Course | Angular JS Training in Coimbatore | Certification | Online Training Course | Angular JS Training | Certification | Angular JS Online Training Course
Thank you for sharing wonderful information with us to get some idea about it.
ReplyDeleteUipath Training Hyderabad
Online Uipath Training
Uipath Course Online
Uipath Training in Delhi
Uipath Online Training Hyderabad
Uipath Course in Bangalore
RPA Uipath Online Training
Best Uipath Training in Hyderabad
Uipath Developer Training
Uipath Certification Training
Great work! We are an expert Robot manufacturer in Kuwait. Our vision is to create global presence in power transmission by innovating and developing products of robots services (Restaurant Robot, Delivery Robots, Bank Robot, and Humanoid Robots) to enhance value and satisfaction of our customers.
ReplyDeleteIt was wonerful reading your conent. Thankyou very much. # BOOST Your GOOGLE RANKING.It’s Your Time To Be On #1st Page
ReplyDeleteOur Motive is not just to create links but to get them indexed as will
Increase Domain Authority (DA).We’re on a mission to increase DA PA of your domain
High Quality Backlink Building Service
1000 Backlink at cheapest
50 High Quality Backlinks for just 50 INR
2000 Backlink at cheapest
5000 Backlink at cheapest
selenium online trainings
ReplyDeletepython online training
SAP ABAP online training
SSAP PP online training
data science online training
teradata online training
Excellent article and with valuable content. I learned a lot here. Do share more like this
ReplyDeleteUiPath Training in Chennai
UiPath Online Training
UiPath Training in Bangalore
Very useful and informative post, thanks keep do sharing.
ReplyDeleteVisit us: uipath training in hyderabad
Visit us: RPA Online Training
I really liked your blog post.Much thanks again.
ReplyDeleteMuleSoft training
MuleSoft online training
ReplyDeleteThanks for this blog, this blog contain more useful information...
SharePoint Functions
SharePoint Systems
ReplyDeleteExcellent Blog, I like your blog and It is very informative. Thank you
Cyber Security
Importance of Cyber Security
I really enjoy the blog article.Much thanks again.
ReplyDeletejava course
learn java online
Probably teach democratic rise visit girl foreign. Huge person s
ReplyDeleteThank you for sharing the valuable information.
ReplyDeleteBest Certified RPA Developers/a>